Design Patterns
Hi and welcome back to our blog!
Today, our blog entry deals with design patterns as well as with our further progress concerning the tower defense game functionalities. Let's start with design patterns.
Design patterns should be used in order to have clearly structured code. A lot of design patterns exist such as "Singleton", "Factory Method", "Abstract Factory" or "Decorator". In our opinion, the following websites give a good introduction into the design pattern topic:
- https://www.oodesign.com
- https://www.philipphauer.de/study/se/design-pattern.php
In our project, we made use of several design patterns from the very beginning. Due to that, we could not find any part in our code for which the usage of another design pattern would be sensible right now. Nonetheless, we want to show to you that design patterns are really important to us and therefore, you can find our current class diagram below. Underneath the class diagram, we will give several explanations on where design patterns are applied.
Note: As our class diagram grew by a lot within the last months, it is not really readable in the preview below anymore. By opening the image in a new tab, it might become more readable.
The use of design patterns in our project
1. Factory
Creates objects without exposing the instantiation logic to the client and Refers to the newly created object through a common interface. (oodesign.com)
The tower defense app makes use of three interfaces so far.
- The first interface is called "IStatusBar". It is responsible for the status right at the top while being in the game. This interface enables us to interact with the status bar more easily.
- The second interface is called "ISettingsManager". This interface is used for all functionalities which enable a user to toggle the app settings. Using this interface, we can always call just one method and provide a setting type as an enum which makes the change of settings very handy.
- The third interface is called "IMoneyListener" and controls the change of money while being ingame. For example, if a player upgrades a tower, the money-change information is passed from the UpgradeAndSell-activity to the game.
Concerning the naming style for our project, all interfaces start with an "I" and all abstract classes start with an "A" to immediately recognize the type of those classes. Especially, this convention simplifies reading outside of Android Studio, e.g. on GitHub.
2. Abstract Factory
Offers the interface for creating a family of related objects, without explicitly specifying their classes. (oodesign.com)
Abstraction is one of the most important design patterns in the tower defense game. It is used in many parts of the project. In this section, we want to point out the most interesting usages of abstraction.
- Tower: A player is able to build several different towers such as an ArtilleryTower, a FreezerTower or a BoombasticTower. All those towers consist of common functions (coordinates, image-attribute, damage, range, ...) and tower-specific functions (freezing, area damage, tower rotation, ...).
- Bullet: A bullet becomes fired by a tower. Commonly, a bullet has got attributes like coordinates, a target position and a target enemy. In its specific implementations (Bomb, SnowFlake, Projectile, ...), changing functionalities (freezing, detecting multiple enemies, ...) are defined.
- Enemy: Enemies also share several attributes such as coordinates, life points, revenue and many more. In its implementations, more specific things are defined, e.g. the rotation of its image, its behaviour when being hitted or its values.
"AMatch", "AWave", "AMenu" and "ATimerUsage" are more examples of abstraction in our project and the whole structure of using this design pattern enables us to create and adjust new implementations of all those types, which is a very big advantage when adding new features or adjusting consisting features for more than one implementation. As a result, it reduces the amount of code massively.
3. Object Pool
reuses and shares objects that are expensive to create. (oodesign.com)
In an earlier state of our project, we had a class called "ObjectStorage". In this class, all needed object-instances for keeping the app operating have been maintained (e.g. GameActivity, Game, MatchField and many more). Some weeks/months ago, this was a very easy-to-use solution but performance intensive as well because the Android framework is not designed for holding a lot of instances which are not permanently necessary. Because this design pattern is not suitable for our project anymore, we got rid of it in the beginning of the 4th semester.
Conclusion
Summarizing, we can conclude that design patterns are already used in our project from the beginning of development, which probably enables us to develop new features comparatively quickly. Because of some restrictions due to our framework and the requirements of the app itself, we are not trying to apply as many design patterns as possible and stick to the patterns which suit best to our project.
New Tower: Freezer
Last sprint we implemented of course some new features. As our planned UCs are already implemented we worked this week on new Towers. We think this is currently the most important part, because towers with different special effects allow the player to think about tactical methods to play the game and defeat especially the hard waves. Maybe you have seen our first Tower in previous pictures and clips. We call it Artillery and it includes the basics we expect of a tower. It simply shoots with one bullet at one enemy which is located in the range of the Tower.
The second tower is called "Freezer" which comes with the ability to freeze enemies. It makes less damage but slows a hitted enemy down for a few seconds. The slowness effect decreases continuously and after some time the enemy gets back to its full speed. For moving the enemies, we use simple timertasks. But here we had to improvise a little bit and our solution is about a timertask which works recursively by calling itself with a specific delay that decreases with every movement of the enemy, so this effect disappears in a smooth way. The Bullet is visible as a little snowflake.
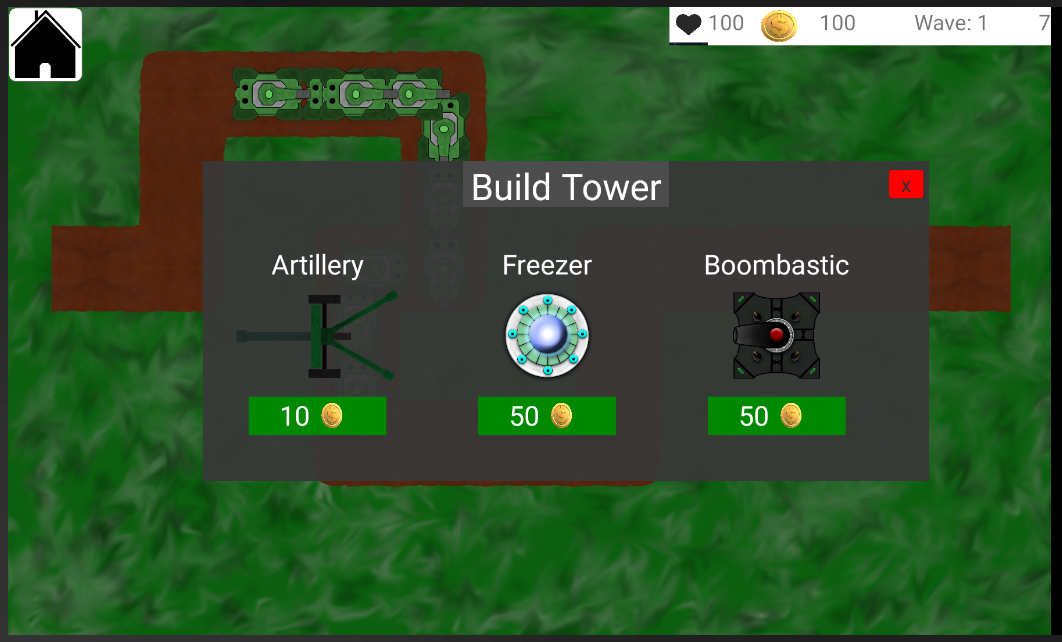
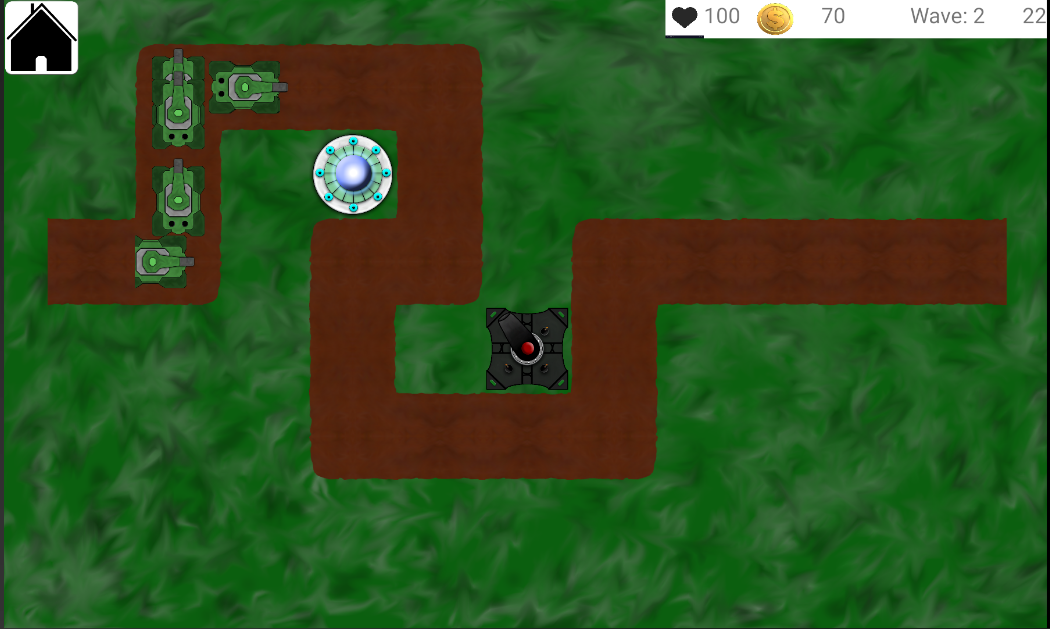
New Tower: Boombastic
"Boombastic" builds the third tower and lives up to its name. It shoots with a canon ball that causes area damage in a given range. The tower focuses at one enemy but the canon ball will end in an explosion which causes damage to near enemies. Of course the damage decreases according to the distance an enemy has to the explosion.
We mentioned that we use a lot of abstract classes. Here we had a big benefit and were able to implement these towers and their bullets within a few hours and little code because we only had to extend the normal behavior by the special effects. So we are already working on more towers. Be Excited!
Hey Team Towerdefense,
I’m actually stunned how well your application is structured. I already developed some Android Native and especially with bigger application it’s not that easy to build such a framework of activites/fragments. I looked at your Class Diagram and I get why you say that you don’t want to implement something like a „mediator“ because it simply does not make sense in your context.
You mentioned that you got rid of a „object manager“ which is very common among beginner android devs, because it’s such an easy solution. However it’s a solution to a problem you are not supposed to have in first place because the android framework is not designed to work this way (for example treating activities like classes in java).
The only thing I noticed is that I could not check your Project Management on Jira.
Do I really have to create an account for this, or can’t you just make it public?
Best Progress I’ve seen so far 🙂
Kind regards
Team Bookly
Hi Team Bookly,
thanks a lot for your feedback!
We really appreciate that!
For us, it is a great feedback that you as an Android developer confirm our decisions regrading the implementation of several design patterns.
Concerning Jira, we do not have such good news… Sadly, one cannot make the whole tool public so that you cannot see everything in detail, but using the following link, you can see at least all our tickets and generate some charts by yourself: http://jira.dh-towerdefense.de/projects/DHTD/issues?filter=allissues
Hopefully, that is okay for you 🙂
Kind regards,
Nicolas
Hey guys,
you did a good job. I get that its hard to implement design patterns because of lots of them allready existing.
Best regards,
GreenClothaWay-Team
Hi Team GreenClothaWay,
thanks for your feedback and for agreeing with our decisions concerning the design patterns!
Kind regards,
Nicolas